Getting Started
The Basics
- What is software? - Software is simply instructions for your computer. It’s the code that you write in order to provide the instructions that different machines run.
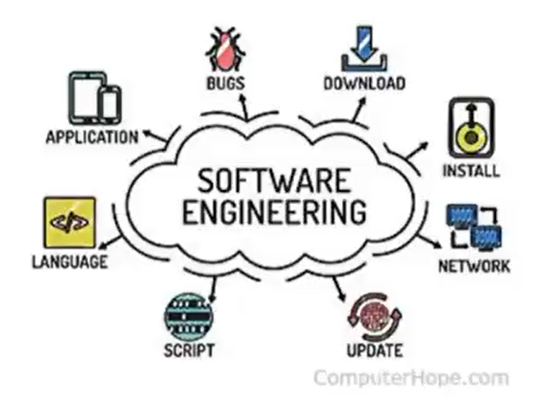
- What is frontend development? - It is the products that are seen by the user. So any of the visual elements that the end user will interact with. A lot of times, this is called your graphical user interface, which you could think of as your webpages or the visual aspects of the applications that you’re using on your devices, such as your iPhone.
- What is backend development? - It is the software aspects that the user doesn’t see. These are the logical elements. So if you want to think of an iceberg, you have what people see on top, the frontend. And then you have the backend, which many times is substantially larger and more complex. Is really all of the structure that supports that frontend, which is the frontend and top of the iceberg, which is really what the users see. Then APIs or application programming interfaces, are the interactions between those two.
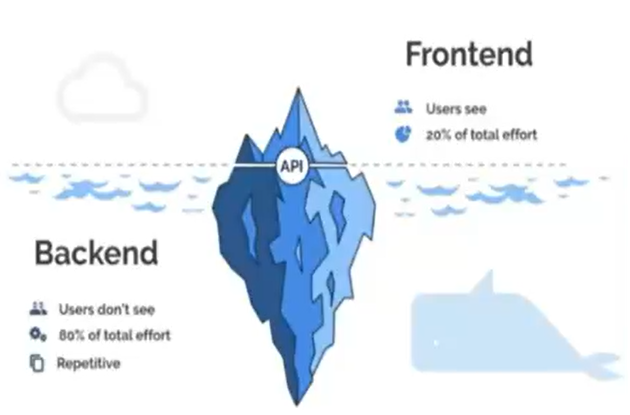
- What is a server? - A server is racks of computers to centralize data function and communication to other devices. This is really where you centralize your compute. But at the end of the day, these are really just computers with compute, whether CPUs or GPUs.
- What is a data center? - A data center is essentially just a large group of connected servers.
- What is an edge device? - Any device, generally client side, outside of the data center. So this is everything from your phones. These are your tablets. These are many times your cars, or it could be an airplane. It could be a fighter. It could be really anything that is sort of disassociated from that server or that data center.
- What is a network? - Its basic level, a network is just a group of computers that are connected.
The Cloud
- Public and Private Clouds? - A public Cloud, is really somebody else’s servers or somebody else’s computers. In a private Cloud, it’s about managing within your organization typically, a set of services that allow people to quickly spin up infrastructure. And so for example, you may have a infrastructure or platform team that manages as a service, your own private Cloud that as a developer within that organization, you can submit tickets or press a button, and next thing, magically, you now have these compute resources. And the biggest difference, private versus public is private, typically self-managed within a single organization, typically the organization who actually owns the servers and then public Clouds would be sort of like your Amazons, your Googles, your Microsoft.
- As a Service - Pizza as a service can be a real life relatable example as shown below,
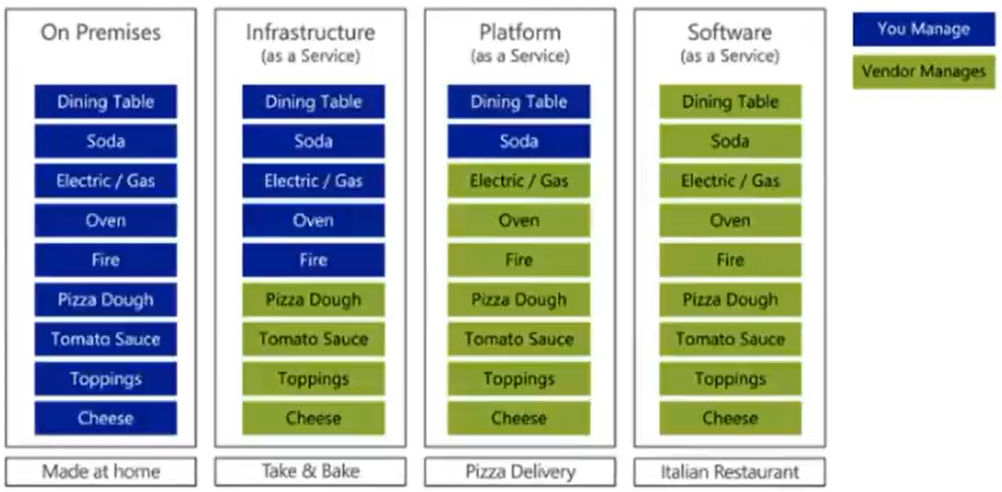
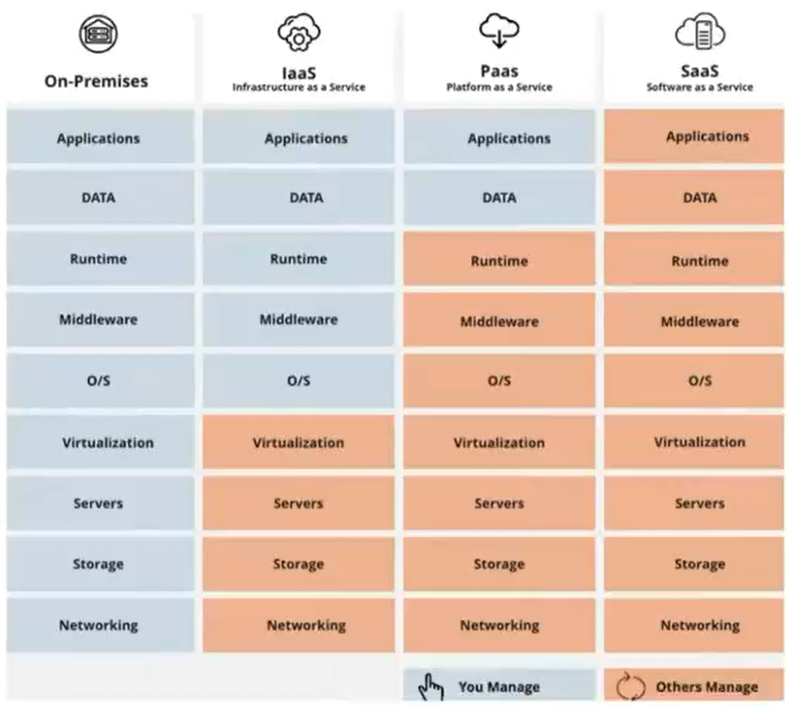
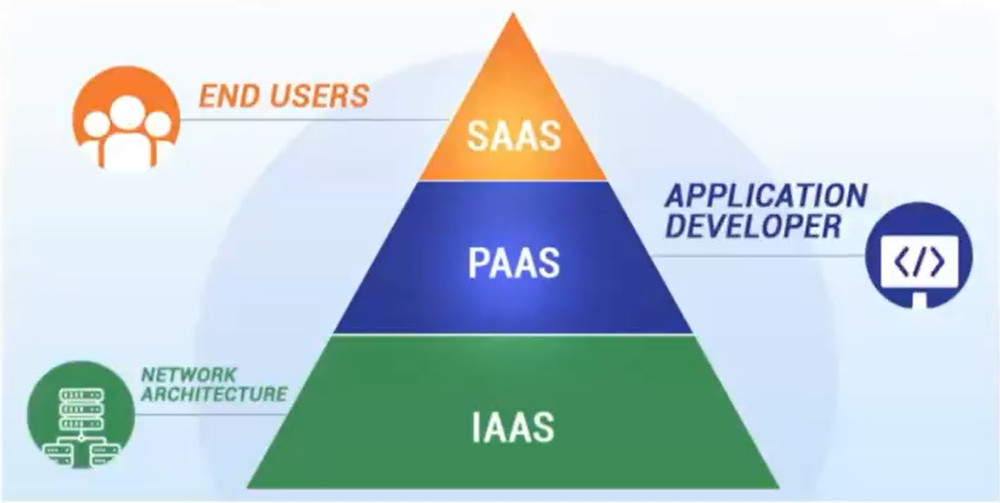
Environments and Pipelines
- What is an environment? - A software environment is simply the logical box, if you will, that you work in that has all of the tools and resources that you need to accomplish your task, whether that’s developing or testing or operating.
- Development Environment
- Quality Assurance Environment
- Staging Environment
- Production Environment
- Disaster Recovery Environment
- What is Source control management? - Source control management is a practice of tracking and managing code changes.
- CI/CD Pipelines
- Continuous Build
- Continuous Integrations
- Continuous Delivery
- Continuous Testing
- Continuous Deployment
- Continuous Operations
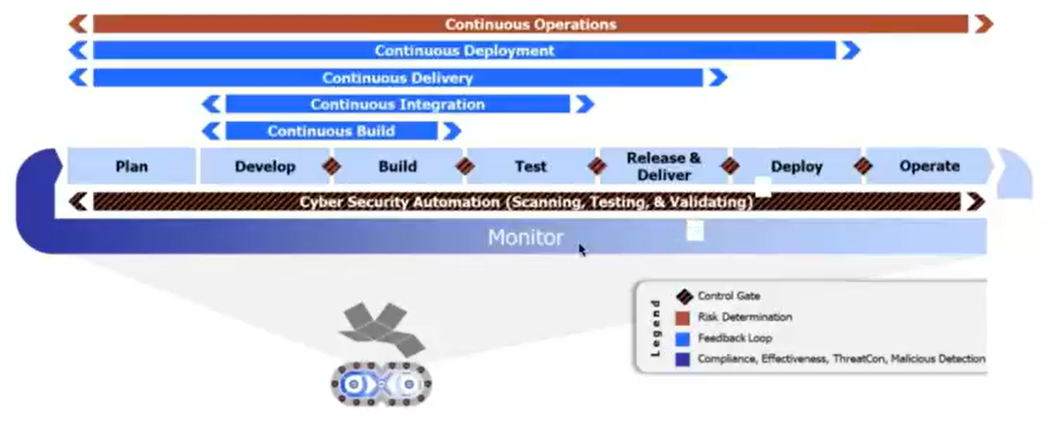
Deployments and Authorizations
- What is imperative and declarative states?
- A declarative state is something that you fully represent and declare - typically in the code base.
- An imperative state is something that you manually go into and configure.
- So, to sort of give you an example of, of how you know when you have a system that’s fully declarative versus something that’s imperative,
is if you have your IT admins or your software engineers interfacing through a CLI in order to customly set up and configure the system.
- The reason why a declarative state is so important is that when you set up things in an imperative way, really only the person who set them up and configured them truly understand and know what the system is.
- Declarative is the code describes what the end state needs to be and then builds it in that fashion. And so, you know, Rob, as you were saying, the only way to truly ensure that you are replicating the environments as desired, the end state, is to use a declarative state. Imperative - there’s room for error, there’s room for issues to arise, and you’re simply following a checklist. You’re not like actually describing the end state and verifying that the end state is what you described it to be.
- why Infrastructure as Code, Configuration as Code, or declarative state ? - This is how do you avoid the situation to where it only works on your machine, but not on somebody else’s.
- So to get started, Infrastructure as Code is the declarative baseline upon which you are establishing your infrastructure to support your software architecture.
And so whether you’re on a cloud environment, or edge or, or on-prem, you can use various technologies: Terraform, Ansible, et cetera, to, to declare how you’re setting up your infrastructure, which once again is very beneficial because you can now, in a repeatable fashion, deploy that same configuration regardless of where you go.
- Mutable and Immutable Infrastructure - if you are using mutable states, there is the opportunity to change those things in production, potentially without even intending to. Versus immutable states, where you know that this needs to stay this way, it should not be reconfigured, or you’re going to essentially like violate the configuration,
violate the baseline, and have now some sort of Delta that is not repeatable back to your other environments. Whereas, you know, immutable would be, okay, these need to stay, this is how we guarantee common baselines across the environments and guarantee stability, reduce that tech debt, reduce the opportunity for fires to occur because something’s changed that didn’t need to change, or shouldn’t have changed.
- GitOps, which is continuously delivering and deploying your declarative baseline through a representation of, effectively, sourced code management and changes.
So if you think about it in terms of source code management within your Git repository, GitOps is how you go from that declarative baseline, the Delta between the new version update, and actually continuously deploying to that production instance.
- Authorization to Operate ATO is essentially your permission to run that software, whether it is infrastructure or platform layer, or application layer software in production on an information system, if you will. So a lot of times we’ll see this in the form of like cybersecurity focus, traditionally, a lot of checklists, and you see this as compliance. It’s really a NIS standard, so it’s highly regulatory environments where this is going to be very common.
- Continuous ATO / C-ATO comes from, depending on really your background and what systems that you’re working on, this concept of a C-ATO might take different flavors.
VMs, Microservices, Containers and Kubernetes
- Why did virtual machines come into existence? - Multiple operating systems on a single set of hardware. So, if you had an application that was Linux-based versus one that is RHEL-based, you could run those without having dedicated hardware for each separate “thing”. So virtual machines virtualize and abstract the infrastructure to allow you to run multiple operating systems on the same infrastructure.
- Prior to virtual machines it was dedicated infrastructure, dedicated operating system, which makes things in many ways more complex. Which is why the community continued to innovate and come out with newer and newer technologies, other than VMs, to make developers' lives easier.
- So here’s sort of an example sort of layout between what it means to have a Virtual Machine. To where you have an application, you have your binaries and libraries, you have that specific operating system, you have a hypervisor, and then you have your actual physical server underneath.
- Why containers came into existence? - It is the next step in abstracting a way that operating system. So, instead of every application having to bring its own operating system, you move to containers where, through the use of something like Docker, you can run a single operating system on top of your hypervisor and then each of the applications or services doesn’t have to bring that. So, they’re lighter weight, if you will.
- Instead of bringing the operating system within your virtualized environment, you’re abstracting it through some type of container engine.
- Why Microservices? - Microservices are the next step of instead of having what we would often refer to as monolithic applications, where everything needing to do that thing, that feature, whatever the application is doing for you, is this single massive chunk of code that’s very interdependent and not really broken up. With microservices, now you start taking out all of the various processes that make up that app.
- Microservices allows you to do is segment your application to smaller chunks, so that way your teams, which many times are decentralized, can all work off a common goal, while not necessarily being fully connected, from sort of a build perspective. You still have to worry about things like end-to-end and integration testing,
because certain microservices are going to rely upon other microservices,
- One of the common terms you’ll hear about containers is this concept called ‘container hardening’, which is very confusing. Like how do you harden a virtual thing? The best way to describe it is just that you’re taking something that exists and you’re ripping things out and making it more secure by minimizing the attack vector. And so many times there might be libraries that aren’t used.
- The concept of container hardening is really predicated, or really hardening anything, is really predicated on the fact that you’re reducing and limiting the “attack surface”. The easiest way to think of an attack surface and attack vector is really no code is fully a hundred percent secure. And so the less code you have and the less areas you have for somebody to go ping and test from an attack vector perspective, the more secure your system is going to be. So, key takeaway here, is that less is more.
- container registries, as essentially your single source of container images, where if you need a specific container to be built somewhere, you would pull from a single container registry. And really, what that lets you do is standardize the way that you interact with that container in the various places that you employ it.
- Kubernetes was really solving that container orchestration problem. And what we mean by that, is that you now have these containers and these microservices, and you’re really trying to solve,
Cybersecurity Technology
- Vulnerabilities - Vulnerability is a known shortcoming from a cybersecurity perspective in the sense that there is a possibility that whatever it is you’re looking at, whether it’s your network or your container, could be taken advantage of, if not patched or fixed or addressed. Vulnerabilities can be both known and unknown.
- Hardening, the point of hardening is to reduce your vulnerabilities. The primary point is to reduce your tax surface to make it so that way you have less vulnerabilities,but a key point here is no system is fully secure.
- All you can do is get better and better and better over time which is why from a cybersecurity technology perspective, it’s very important that you have multiple layers of security, because at any moment in time, there might be an unknown vulnerability that somebody can be actively exploiting or even a known vulnerability that has sort of published a new way to patch it, yet in the, out in what’s known quote in the wild, somebody is actively exploiting that vulnerability.
- From a conceptual level, the best way to protect your system in many ways is to just ensure that the people who shouldn’t have access can’t get in and the people who should are able to. However, that’s not always possible, which is why you have newer technologies that are constantly evolving, constantly innovating. One of which are service mesh technologies.
- What zero trust is? - zero trust as simply stating that there’s no inherited authentication. So you don’t get in and then automatically have access to multiple things.
Everywhere that you go, you have to reauthenticate including point-to-point authentication, even machine to machine. So non-person entities have to authenticate between each other before they can transmit information. Zero trust just goes down to how fine of a level of security and trust you provide really once somebody is trying to gain access to anything, whether it be data or a network.
- software bill of materials SBOM - If you place a large order and you get a box, you’ll get an invoice that lists everything that’s in that order, right? It’s your bill of materials. So a software bill of materials is the same thing. When you have a software package that contains multiple things, multiple containers, libraries,
et cetera, et cetera, you now have a bill of materials that indicates what’s in that package so that at all times you know what you have from a software perspective.
It is especially important when you start looking at dependencies being packaged with software. So you may think you’re getting X, but it needs Y and Z to go with it, that may not be readily apparent at face value, but with the software bill of materials, now you can track down to that level exactly what’s running on your system.
- continuous runtime monitoring - Even though we have all of these layers of security applied, if we’re not actually watching and monitoring those, both to make sure that they’re operating as intended, but also that, going back to unknown vulnerabilities, that something that’s not supposed to happen isn’t happening.
And if you’re not watching, or monitoring in this case, how will you know that? Right? And so you want be able to have that continuous monitoring data, both from an alerting perspective, which I think we’ll get into, but also from a logging perspective, which I think we’ll also get into to be able to identify and respond appropriately. This is comprised of two components continuous logging and continuous alerting
Reference: https://learning.edx.org/course/course-v1:LinuxFoundationX+LFS180x+2T2022/home